Masonry
The Masonry component is a useful tool for creating responsive grid layouts with varying column widths. It is highly customizable with the breakpointCols
, className
, and columnClassName
props, and can be easily integrated into any React project.
Installation
npm install @madeinhaus/masonry
Import
import Masonry from '@madeinhaus/masonry';
import '@madeinhaus/masonry/dist/index.css';
Props
breakpointCols
: an object that specifies the number of columns to be used at various breakpoints. This prop is optional and defaults to{ default: 2 }
.className
: a string that allows for adding a CSS class to the root element of the component.columnClassName
: a string that allows for adding a CSS class to the column elements of the component.children
: a required prop that represents the child elements to be rendered in the Masonry component.
Usage
MasonryDemoBasic.tsx
import Masonry from '@madeinhaus/masonry';
import styles from './MasonryDemo.module.css';
const MasonryDemoBasic: React.FC = ({ images }: { images: string[] }) => {
return (
<Masonry
breakpointCols={{
default: 2,
768: 3,
1024: 4,
}}
>
{images.map((src, index) => {
return (
<figure key={index} className={styles.item}>
<img src={src} />
<figcaption className={styles.caption}>
{index + 1} <br />
</figcaption>
</figure>
);
})}
</Masonry>
);
};
export default MasonryDemoBasic;
MasonryDemo.module.css
.item {
position: relative;
}
.caption {
position: absolute;
top: 0;
left: 0;
color: #e600e6;
background-color: #fff0ff;
padding: 0.5rem;
border-bottom-right-radius: 0.5rem;
}
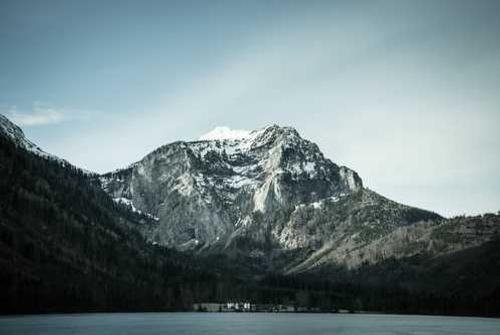
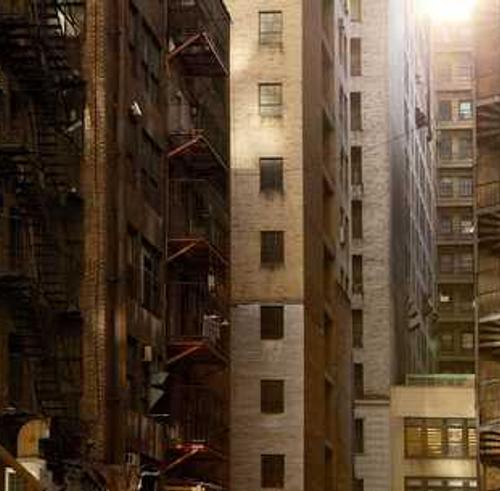
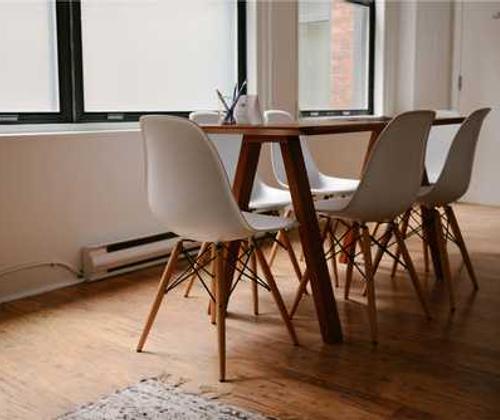
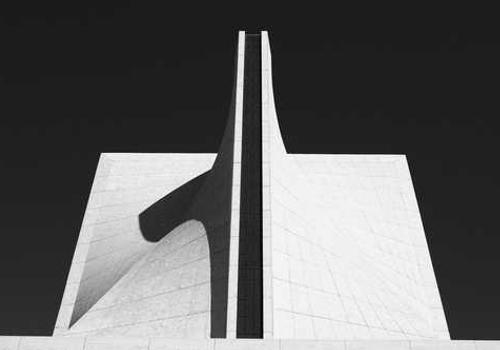
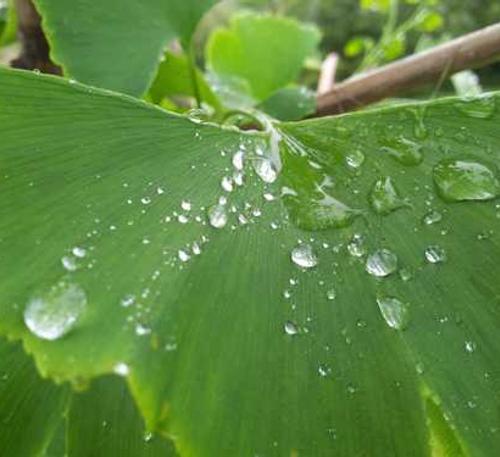
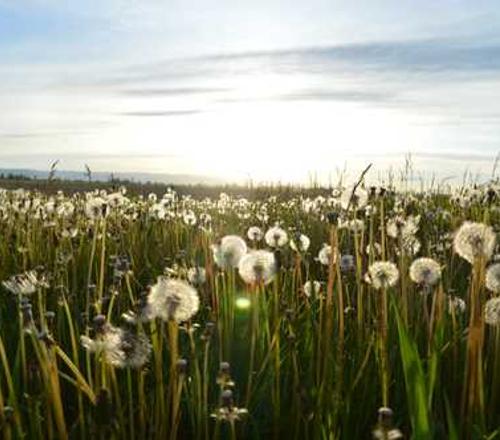
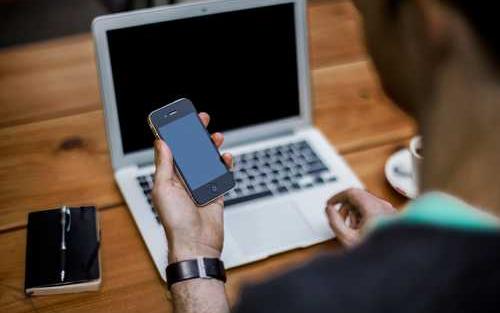
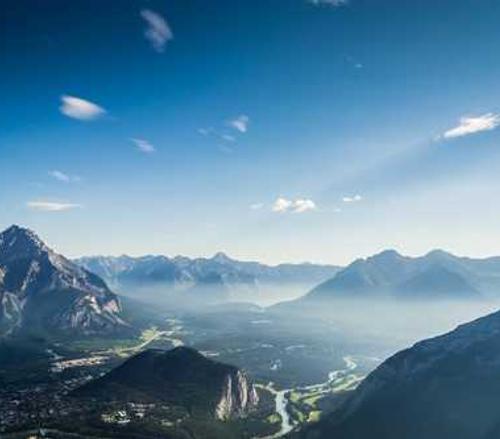
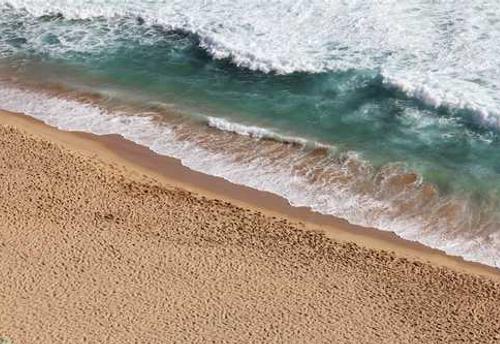
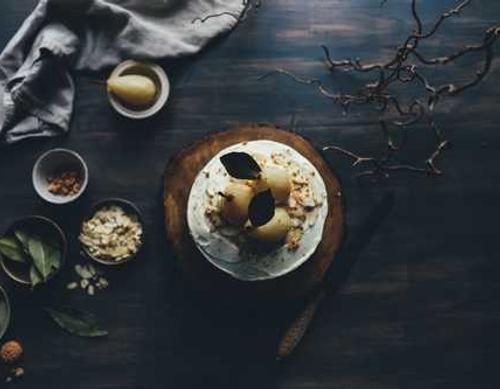
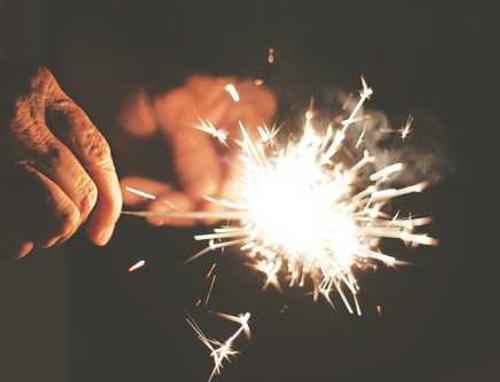
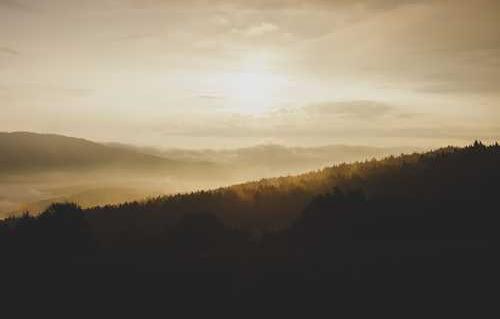
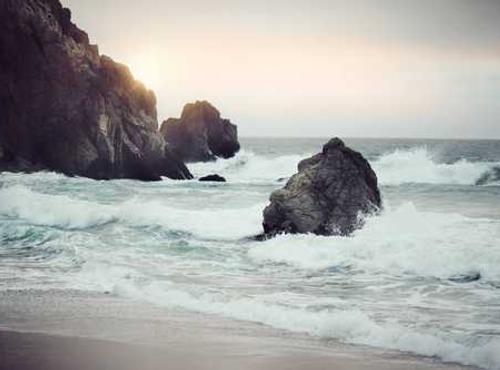
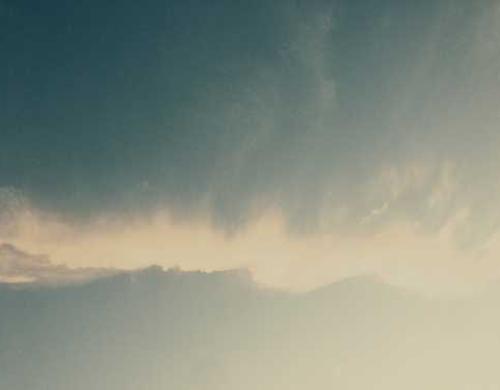
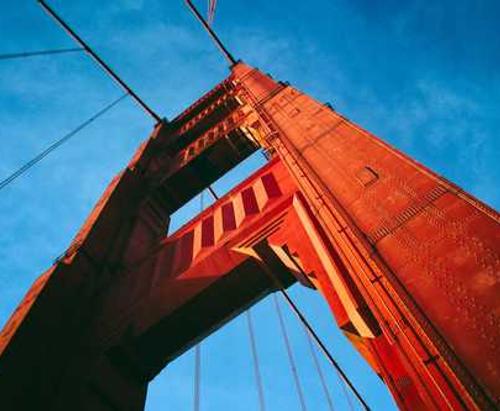
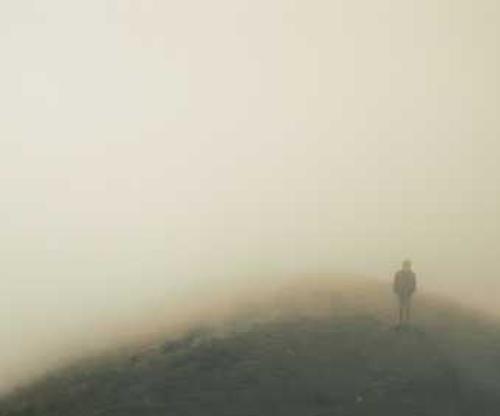

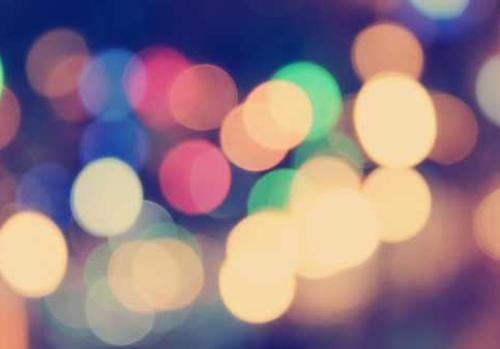
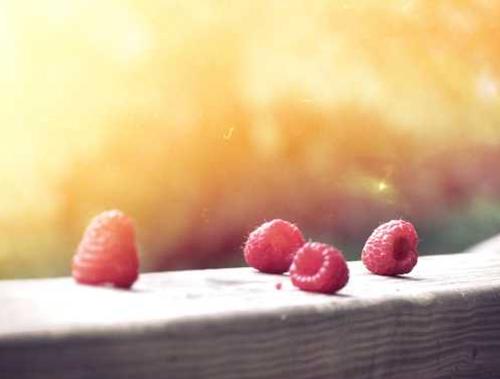
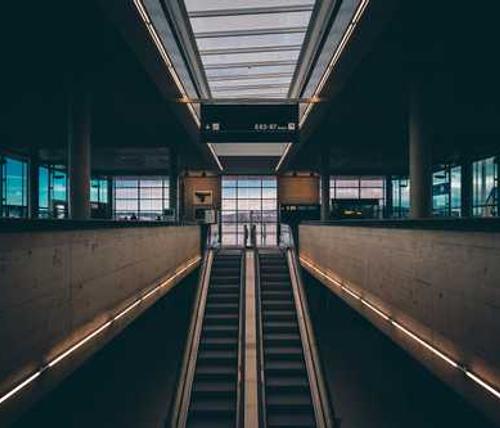